Table of Contents
Introduction:
To get Job in Software industry or IT industry you need to know about logical programs and pattern programs. This Programs questions majority of times decide whether you will get a job or not. I will say Logical Programs are highly important to crack interviews.
In this article I will share with you Top 15 logical programs that will be asked in interviews or any kind of test.
Top 10 Logical Programs:
- What is Armstrong Number? write a code to check number is Armstrong or not.
Definition: Sum of cube of each digit is equal to original number is called as Armstrong number.
Example: 1,153, 371, 407.
153= (1^3)+(5^3)+(3^3)=1+125+27=153
(I have written code in Java)
public class Code{
public void method1()
{
int num=153,rem,sum=0,num1=num;
while(num>0)
{
rem=num%10;
num=num/10;
sum=(rem*rem*rem)+sum;
}
if(num1==sum)
System.out.println(num1 + ” is Armstrong number”);
else
System.out.println(num1 + ” is not an Armstrong number”);
}
public static void main(String [] args)
{
Code ab = new Code();
ab.method1();
}
}
2. What is Fibonacci series? write a code.
Definition: Fibonacci sequence is a sequence in which each number is the sum of the two preceding ones.
Example: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144
public class Code{
public void method1()
{
int num=0,num1=1,sum=0;
System.out.print(num +”, ” + num1 + “, “);
for(int i=0;i<=10;i++)
{
System.out.print(sum + “, “);
sum=num+num1;
num=num1;
num=sum;
}
}
public static void main(String [] args)
{
Code ab = new Code();
ab.method1();
}
}
3. What is Palindrome Number? write code to check number is P.N. or not.
Definition: When we reverse the original number then that number becomes same as original is known as palindrome number.
Example: 1234321 –> when reversed –>1234321 are called as Palindrome number.
123454, 45657, 4532 are examples of non palindrome number.
public class Code{
public void method1()
{
int num=242,rem,sum=0,num1=num;
while(num>0)
{
rem=num%10;
num=num/10;
sum=(sum*10)+rem;
}
if(num1==sum)
System.out.println(num1 + ” is Palindrome number”);
else
System.out.println(num1 + ” is not a Palindrome number”);
}
public static void main(String [] args)
{
Code ab = new Code();
ab.method1();
}
}
4. What is Prime number? write code to check prime number.
Definition: A number which is divisible by 1 and number itself then that number is called as Prime number.
Example: 2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53
public class Code{
public void method1()
{
int num=23,count=0;
for(int i=2;i<num;i++)
{
if(num%i==0)
count++;
}
if(count==0)
System.out.print(num + ” is a Prime number”);
else
System.out.print(num + ” is not a prime number”);
}
public static void main(String [] args)
{
Code ab = new Code();
ab.method1();
}
}
5. What is Palindrome String? write code.
Definition: when we reverse a string then that string becomes original String is known as Palindrome string.
Example: “dad”,”mom”, “madam” —> “madam” is a Palindrome String.
public class Code{
public void method1()
{
String str =”madam”; String revStr = “”;
for(int i=0;i<str.length();i++)
{
char ch = str.charAt(i);
revStr = ch + revStr;
}
if(str.equals(revStr))
System.out.println(str + ” is a Palindrome String”);
else
System.out.println(str + ” is not a Palindrome String”);
}
public static void main(String [] args)
{
Code ab = new Code();
ab.method1();
}
}
6. Sort Array without using sort method.
To sort array we have one method Arrays.sort() —> using this method we can sort array but we have to sort without using this method.
public class Code{
public void method1()
{
int a[] = {5, 6, 4, 2, 1, 3, 8, 7};
for(int i=1;i<=10;i++)
{
for(int j=0;j<a.length;j++)
{
if(i==a[j])
System.out.print(a[j] + ” “);
}
}
}
public static void main(String [] args)
{
Code ab = new Code();
ab.method1();
}
}
7. Find Maximum and Minimum value from array also find index number of max & min value.
public class Code{
public void maxValue()
{
int a[] = {5, 6, 4, 2, 1, 3, 8, 7};
int max = a[0]; int index=0;
for(int j=0;j<a.length;j++)
{
if(a[j]>=max)
max=a[j];
if(a[j]>=max)
index =j;
}
System.out.println(“Maximum value in array : ” + max);
System.out.println(“Maximum value index number : ” + index);
}
public void minValue()
{
int a[] = {5, 6, 4, 2, 1, 3, 8, 7};
int min = a[0]; int index=0;
for(int j=0;j<a.length;j++)
{
if(a[j]<=min)
min=a[j];
if(a[j]<=min)
index =j;
}
System.out.println(“Minimum value in array : ” + min);
System.out.println(“Minimum value index number : ” + index);
}
public static void main(String [] args)
{
Code ab = new Code();
ab.maxValue();
ab.minValue();
}
}
8. Write a code for given Patterns?
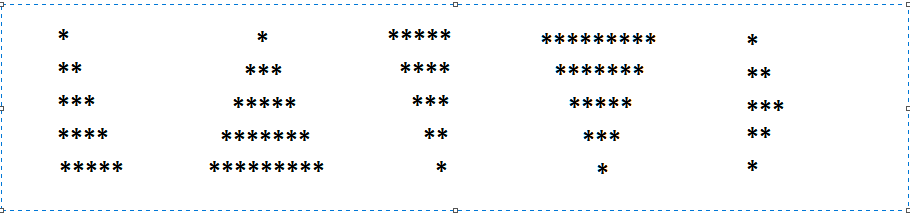
In interview you will be given one pattern and you have to write a code for that pattern.
Selenium Java Important interview questions definition of String array, constructor,